In today's digital age, Application Programming Interfaces (APIs) have become an essential part of software development. An API allows external systems to communicate with your system and execute its functions remotely, providing a way for clients to use your system's functionalities. However, creating a good API is not an easy task. In this blog post, I'll explore the characteristics of four types of APIs, categorizing them based on access level, protocol, and data type. I'll also discuss the pros and cons of each architectural style.
Now, you might be wondering what makes your API great. Well, here are some key things to keep in mind:
- Simplicity - an API should require as few input parameters as possible to get the job done. It should have one main responsibility and do it well. There is no need for unnecessary complexity here.
- Transparency - an API should be like a reliable friend that you can count on to deliver the expected results. You want to be able to predict what it will do with ease.
- Purity - the API should always give the same output for the same input.
- Clarity - all exposed functions and methods should be clearly documented in a way that's easy for external developers to understand. It's like having a helpful guide that explains everything in detail. You want to make sure they can use your API with confidence.
How we can divide an APIs
In the beginning, it's worth understanding how we can divide APIs.
The API can be divided into groups by intended level of access, the way data is transferred, and the type of the transferred data. Each type of API has different characteristics, such as availability, security, scalability, and backward compatibility.

Division due to the access level
Not all API’s are accessible to every type of client. Some API’s are designated for internal use only, while others are open to the public. Additionally, there are API’s specifically for authorized partners. Understanding and managing these access levels is essential for maintaining security and functionality within your system. We can list 4 types of the API’s divided due to their access level: public, private, partner and composite. The following table shows the characteristics of all of them.
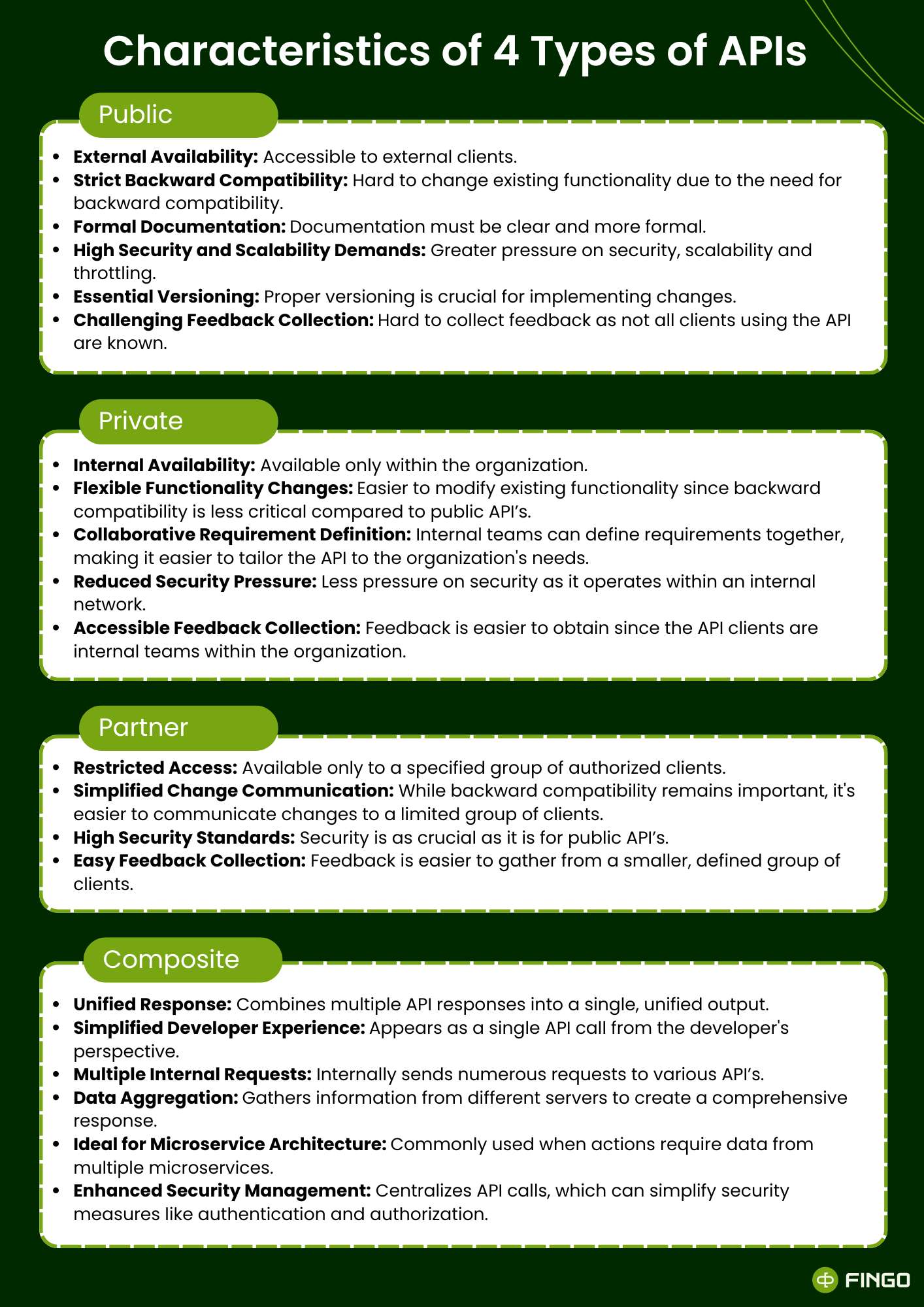
Division due to the architectural styles
There are many API architectural styles, each with its own pros and cons. Some styles are ideal for certain systems, while others may not be as effective.
Understanding your system's needs will help you choose the best architectural style for optimal performance and compatibility.
REST
REST (Representational State Transfer) is the most renowned architectural style for APIs, widely implemented across hypermedia distributed systems. REST isn't just a protocol, it's an architectural style defined by a specific set of constraints designed to enhance system performance, scalability, and simplicity.
Pros of REST
- Robustness: ensures reliable and stable system performance.
- Scalability: facilitates the growth and scaling of systems to handle increasing loads.
- Maintainability: promotes easier maintenance and updates of the system.
- Adaptability: enables systems to evolve and adapt to changing requirements over time.
- Clear Principles: provides a well-defined set of principles that guide API design and development.
Cons of REST
- Complex Implementation: requires understanding and implementing multiple constraints which can be complex.
- Statelessness Limitation: stateless interactions can lead to increased overheads in each request, as all contexts must be included.
- Caching Challenges: properly indicating cache-ability requires careful planning and implementation.
- Uniform Interface Rigidity: adhering to a uniform interface may limit flexibility in some cases.
- Optional Code-On-Demand: the optional nature of code-on-demand can lead to inconsistent implementation and usage.
RPC
RPC (Remote Procedure Call) aims to simplify communication between distributed systems by allowing procedures/functions to run on remote computers as if they were local. This abstraction hides the complexity of communication, enabling programmers to focus on coding rather than handling communication protocols.
Key Implementations of RPC:
- XML-RPC
- JSON-RPC
- gRPC
Pros of RPC
- Local-like External Calls: enables calling external procedures as if they were local simplifying the development process.
- Simplified Distributed Systems: makes it easier to build and manage distributed systems by abstracting communication details.
Cons of RPC
- Complex XML Implementation: XML-RPC can be complex and add latency to package transmission.
- Latency Concerns: JSON-RPC and gRPC offer better performance but can still face latency issues if not properly optimized.
SOAP
One year after the release of XML-RPC, Microsoft introduced SOAP (Simple Object Access Protocol). Built on the XML-RPC concept, SOAP offers greater flexibility by allowing the transfer of complex objects rather than just simple data types.
Pros of SOAP
- Simplifies Distributed Systems: facilitates the development and management of distributed systems.
- Complex Object Transfer: allows sending complex objects, not just simple data types.
- Built-in Security: defines its own security mechanism (WS-Security), enhancing data protection.
Cons of SOAP
- Increased Complexity: the implementation is more complex than RPC due to the capability of sending objects.
- Packet Size and Latency: being based on XML, it suffers from larger packet sizes, which can add latency to data transmission.
GraphQL
GraphQL is one of the newest API concepts described as a query language rather than an architectural style. Its declarative style provides programmers with significant flexibility for fetching and mutating data. By allowing clients to request only the data they need, GraphQL prevents over-fetching, unlike REST API’s in which the server dictates the response content.
Pros of GraphQL
- Flexible Queries: clients can request exactly the data they need, avoiding over-fetching.
- Strongly Typed Schema: provides a clear, structured schema that enhances development and debugging.
- Efficient Data Retrieval: allows for efficient data retrieval, particularly for simple queries.
Cons of GraphQL
- Complexity: involves learning a new query language and complex configuration.
- Server Overhead: can introduce additional server overhead due to the need to resolve complex queries.
- Performance Issues: complex, nested queries can lead to slow data retrieval.
Division due to data format
A very important part of API is the data. Not what data is sent but what the structure of the data is and what benefits that structure gives. Nowadays the two most common data types used in the vast majority of API’s are JSON and the XML.
JSON
JSON stands for JavaScript Object Notation. It’s simple, lightweight data format that allows you to transfer objects and consists of key-value pairs. JSON Supports just a few basic data types: Number, String, Boolean, Array, Object and null.
Pros of JSON
- Simplicity: JSON is straight-forward and easy to understand, making it accessible for developers of all levels.
- Lightweight: its minimal syntax results in smaller file sizes compared to other data formats, optimizing network bandwidth and storage space.
- Flexible Data Structure: JSON supports a variety of basic data types including numbers, strings, booleans, arrays, objects, and null values, offering versatility in data representation.
Cons of JSON
- Limited Data Types: JSON supports only a limited set of basic data types, which can be restrictive for certain use cases requiring more complex data structures.
- Lack of Schema Definition: unlike XML, JSON does not provide built-in support for defining a schema, which can lead to issues with data validation and interoperability in larger systems.
- No Comments: JSON does not support comments within the data structure, making it challenging to document or annotate the data for future reference or clarification.
XML
XML (Extensible Markup Language) is the data format that allows you to transfer more complex objects and consists of the same types as JSON and additional types like dates, images and namespaces.
Pros of XML
- Supports Complex Data: XML can handle complex data structures, including additional types like dates and images.
- Hierarchical Structure: Its hierarchical structure aids in organizing and categorizing data effectively.
- Schema Definition: XML supports schema languages for data validation, ensuring integrity and interoperability.
Cons of XML
- Verbose Syntax: XML's verbosity leads to larger file sizes compared to JSON.
- Complexity: managing large XML schemas can become complex and challenging.
- Parsing Overhead: parsing XML documents can be resource-intensive, impacting performance in high-throughput systems.
Conclusion
In conclusion, there are numerous types of API’s, each differing in architectural style, access level, protocol, and data format. Choosing the right API for your organization depends largely on your business needs and the specific purposes for which the system was created. Each type of API has its strengths and is best suited for particular use cases. Therefore, it is crucial to conduct thorough research and planning before starting implementation. By doing so, you can ensure that your API meets all your requirements and effectively supports your business goals. Suitable preparation will help you avoid the pitfalls of an ill-fitting API and ensure the successful integration for your company.
References
• Ruupert Koponen: Understanding re-emergence of the RPC model in web development (Aalto University BACHELOR’S THESIS 2024)
• Anshu Soni, Virender Ranga: API Features Individualizing of Web Services: REST and SOAP
• https://ics.uci.edu/~fielding/pubs/dissertation/rest_arch_style.htm